Use React Native to create mobile applications. Discover the benefits and features of React Native and learn how to start building your own apps today.
React Native is a modern, efficient platform for creating mobile applications. Whether you're an experienced developer or simply curious to discover the world of app development, React Native is a powerful tool at your disposal. Using this technology, you'll be able to design apps for iOS and Android using a single source code. This means you'll save time and effort, while delivering a fluid, responsive user experience. In this article, we'll explore the benefits and features of React Native in detail, and show you how to start building your own mobile apps today. Whether you're a freelance developer or a company looking for a rapid development solution, React Native is the ideal option for your needs.
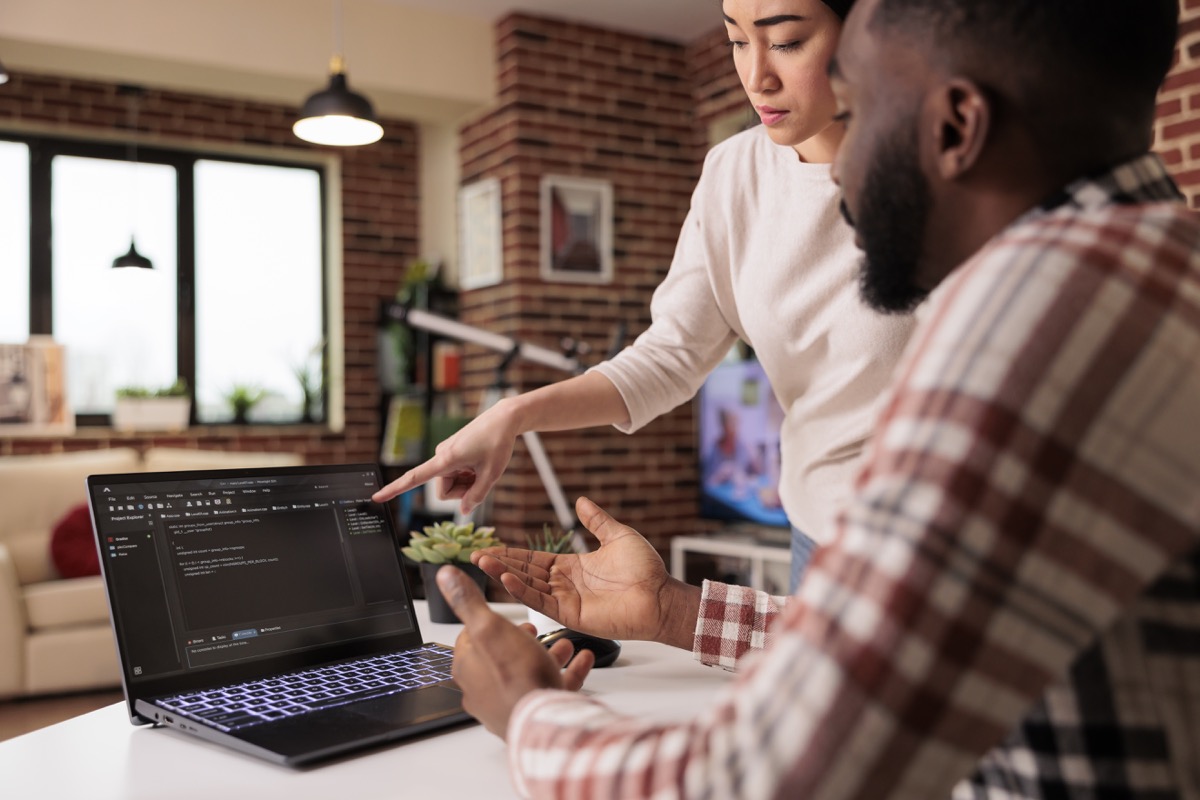
What is React Native?
React Native is an open source framework developed by Facebook to create cross-platform mobile applications. The main difference between React Native and other frameworks of mobile application development such as Cordova or Xamarin, is that React Native doesn't create Web applications packaged inside a native application, but rather truly native applications.
History
React Native first appeared in 2015 and quickly gained popularity due to its simplicity and flexibility for creating mobile apps. It is based on React, a JavaScript framework used to develop user interfaces interactive applications. Using the same concept of reusable components, React Native enables developers to create mobile applications for iOS and Android using a single source code.
Basic principles
The basic principles of React Native are similar to those of React. It is based on the creation of reusable components that can be combined to build a user interface. Each component is an autonomous part of the UI that can have its own internal state. When the state of a component changes, React Native automatically updates the user interface to reflect these changes.
Benefits
React Native offers many advantages for mobile application developers. Firstly, it reduces development time by sharing code between different platforms. Instead of separately developing an app for iOS and another for Android, developers can use the same code for both platforms.
What's more, React Native maintains the performance and appearance of a native application, as it uses the native components of the underlying platforms. This means that applications developed with React Native run smoothly and look consistent across different platforms.
Finally, React Native benefits from a large community of developers, offering numerous tutorials, libraries and additional resources to facilitate the development of mobile applications.
Installing React Native
Prerequisites
Before installing React Native, there are a few prerequisites to consider. First, make sure you have Node.js installed on your machine. Node.js is required to run the Node.js package manager, npm, which is used to install and manage React Native dependencies. You'll also need a text editor or a IDE to write your code.
Installation on macOS
To install React Native on macOS, you can use npm by executing the following command in your terminal:
npm install -g react-native-cli
This command installs the React Native CLI globally. Once the installation is complete, you can create a new React Native project by running the following command:
react-native init MyReactNativeProject
Installation on Windows
To install React Native on Windows, you can use Chocolatey, a package manager for Windows. First, open a command prompt as administrator and run the following command to install Chocolatey:
choco install -y nodejs.install python2 jdk8
Next, you can use npm to install the React Native CLI by running the following command:
npm install -g react-native-cli
Installation on Linux
To install React Native on Linux, you can use npm by executing the following commands in your terminal:
sudo apt-get update
sudo apt-get install -y curl
curl -sL https://deb.nodesource.com/setup_12.x | sudo -E bash -
sudo apt-get install -y nodejs
sudo apt-get install -y build-essential
sudo apt-get install -y git
Next, you can install the React Native CLI with npm by running the following command:
npm install -g react-native-cli
Getting started with React Native
Creating a React Native project
Once you've installed React Native, you can create a new project using the React Native CLI. Open your terminal and run the following command:
react-native init MyReactNativeProject
This will create a new directory containing all the files required for your React Native project. Once creation is complete, you can navigate to your project directory using the following command:
cd MyReactNativeProject
Structure of a React Native project
The structure of a React Native project is similar to that of a React application. The application's entry point is generally found in a file called App.js
directory, where you can define your root component. The other files and directories in the project are used to organize and manage the various components, styles, images and so on.
Running a React Native application
To run your React Native application, open your terminal and make sure you're in your project directory. Then run the following command:
react-native run-ios
or
react-native run-android
This will launch the React Native packager and build your app on the iOS or Android simulator, depending on the command you used. You can then watch your application run on the simulator.
Basic React Native components
View
The component View
is the equivalent of a div container in React Native. It can be used to organize and group other components, and can also be styled with custom CSS properties.
import React from 'react';
import { View } from 'react-native';
const App = () => {
return (
{/* view content */}
);
}
export default App;
Text
The component Text
is used to display text in a React Native application. It uses native text styles and can also be styled with custom CSS properties.
import React from 'react';
import { View, Text } from 'react-native';
const App = () => {
return (
Hello world!
);
}
export default App;
Image
The component Image
allows you to display images in a React Native application. It can load local or remote images and supports automatic image caching.
import React from 'react';
import { View, Image } from 'react-native';
const App = () => {
return (
);
}
export default App;
TextInput
The component TextInput
allows users to enter text in a React Native application. It supports various native keyboard types and can be customized with CSS properties.
import React, { useState } from 'react';
import { View, TextInput, Button } from 'react-native';
const App = () => {
const [text, setText] = useState('');
const handleInputChange = (value) => {
setText(value);
}
const handleButtonPress = () => {
alert(`You have entered: ${text}`);
}
return (
);
}
export default App;
Button
The component Button
is used to create a clickable button in a React Native application. It can be customized with CSS properties and associated with a callback function that will be executed when the button is pressed.
import React from 'react';
import { View, Button } from 'react-native';
const App = () => {
const handleButtonPress = () => {
alert('The button has been clicked');
}
return (
);
}
export default App;
Styles in React Native
Using online styles
In React Native, styles can be defined directly inside components using style properties. Styles are defined using a syntax similar to CSS inline style sheets.
import React from 'react';
import { View } from 'react-native';
const App = () => {
return (
{/* view content */}
);
}
export default App;
Using external style sheets
It is also possible to define styles in external style sheets using the StyleSheet
from React Native.
import React from 'react';
import { View, StyleSheet } from 'react-native';
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
const App = () => {
return (
{/* view content */}
);
}
export default App;
Dynamic style management
To manage dynamic styles in React Native, you can use state variables or computed properties to modify styles according to context or user interaction.
import React, { useState } from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
const [isToggled, setToggled] = useState(false);
const handleButtonPress = () => {
setToggled(!isToggled);
}
const dynamicStyle = isToggled ? styles.containerActive : styles.containerInactive;
return (
The style changes depending on the button
);
}
const styles = StyleSheet.create({
containerActive: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'green',
},
containerInactive: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'red',
},
text: {
fontSize: 24,
fontWeight: 'bold',
color: 'white',
},
});
export default App;
Interaction with users
Event management
Event management in React Native is similar to that in React. You can add event listeners to components and execute callback functions when events occur.
import React from 'react';
import { View, Button } from 'react-native';
const App = () => {
const handleButtonPress = () => {
alert('The button has been clicked');
}
return (
);
}
export default App;
Using gestures
React Native also detects and reacts to user gestures, such as swiping, pinching and rotating. You can use specific React Native gesture components to add these features to your application.
import React, { useState } from 'react';
import { View, StyleSheet, PanResponder } from 'react-native';
const App = () => {
const [position, setPosition] = useState({ x: 0, y: 0 });
const handlePanResponderMove = (event, gestureState) => {
setPosition({ x: gestureState.dx, y: gestureState.dy });
}
const panResponder = PanResponder.create({
onStartShouldSetPanResponder: () => true,
onPanResponderMove: handlePanResponderMove,
});
return (
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
box: {
width: 100,
height: 100,
backgroundColor: 'red',
},
});
export default App;
Element animation
React Native offers tools for creating fluid, interactive animations in your applications. You can use React Native's animation components and animation APIs to animate component properties.
import React, { useState, useEffect } from 'react';
import { View, Animated, Easing } from 'react-native';
const App = () => {
const [opacity] = useState(new Animated.Value(0));
useEffect(() => {
Animated.timing(opacity, {
toValue: 1,
duration: 1000,
easing: Easing.linear,
useNativeDriver: true,
}).start();
}, []);
return (
Hello world!
);
}
export default App;
Navigating a React Native application
Using React Navigation
React Navigation is a popular library for managing navigation between screens in a React Native application. It offers predefined navigation components such as StackNavigator
, TabNavigator
and DrawerNavigator
to make navigation easier.
import React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './screens/HomeScreen';
import DetailScreen from './screens/DetailScreen';
const Stack = createStackNavigator();
const App = () => {
return (
);
}
export default App;
Route creation
To create routes in a React Native application, you can use the React Navigation components. Each route corresponds to a screen in the application and can be configured with additional properties such as parameters or navigation options.
import React from 'react';
import { View, Text, Button } from 'react-native';
const HomeScreen = ({ navigation }) => {
const handleButtonPress = () => {
navigation.navigate('Detail', { id: 1 });
}
return (
Home page
);
}
export default HomeScreen;
Passing parameters between routes
To pass parameters between routes in a React Native application, you can use the React Navigation options. Parameters can be passed when navigating from one route to another, and can be retrieved in the destination component.
import React from 'react';
import { View, Text } from 'react-native';
const DetailScreen = ({ route }) => {
const { id } = route.params;
return (
Detail of element {id}
);
}
export default DetailScreen;
Data management in React Native
Using the Fetch API for HTTP requests
React Native offers a Fetch API similar to that of web browsers for making HTTP requests. You can use it to send and receive data from a server.
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
const App = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(jsonData => setData(jsonData))
.catch(error => console.log(error));
}, []);
return (
{data ? (
{data.message}
) : (
Loading data...
)}
);
}
export default App;
Using AsyncStorage for local storage
React Native also offers a module called AsyncStorage for asynchronous data storage, similar to local storage in web browsers. You can use it to store data such as authentication tokens or user preferences locally on the device.
import React, { useEffect, useState } from 'react';
import { View, Text, Button } from 'react-native';
import AsyncStorage from '@react-native-async-storage/async-storage';
const App = () => {
const [data, setData] = useState(null);
useEffect(() => {
AsyncStorage.getItem('data')
.then(storedData => {
if (storedData) {
setData(storedData);
}
})
.catch(error => console.log(error));
}, []);
const handleButtonPress = () => {
const newData = 'New data';
AsyncStorage.setItem('data', newData)
.then(() => setData(newData))
.catch(error => console.log(error));
}
return (
{data ? (
{data}
) : (
No data found
)}
);
}
export default App;
Using Redux for global state management
To manage the global state of a React Native application, you can use Redux, a predictable state management library. Redux lets you centralize application state in a single location and make it accessible from any component of the application.
import React from 'react';
import { View, Text, Button } from 'react-native';
import { connect, useDispatch, useSelector } from 'react-redux';
import { incrementCounter, decrementCounter } from './actions/counterActions';
const App = () => {
const counter = useSelector(state => state.counter);
const dispatch = useDispatch();
const handleIncrement = () => {
dispatch(incrementCounter());
}
const handleDecrement = () => {
dispatch(decrementCounter());
}
return (
{counter}
);
}
export default connect()(App);
Testing and debugging in React Native
Using the Chrome debugger
React Native offers a debugging feature that connects the application to the Chrome development tool. This makes it possible to debug and inspect the code. JavaScript of the application directly from the Chrome browser.
To use the Chrome debugger, you must first enable USB debugging on your Android device or emulator. Then run your React Native application with the following command:
react-native run-android
Once the application has been launched on your device or emulator, open Google Chrome and enter chrome://inspect
in the address bar. You should see your React Native application listed in the "Devices" panel. Click on the "Inspect" button to open the Chrome development tools.
Using integrated debugging tools
In addition to the Chrome debugger, React Native also offers integrated debugging tools that can be used directly in the emulator or on the device.
For example, you can use the console.log
to display debugging messages in the terminal console or in the Expo application's Developer panel. You can also use the Element Inspector to inspect your application's elements and modify their style on the fly.
To activate the element inspector in the iOS emulator, press Cmd + D
. For the Android emulator, press Cmd + M
or shake your physical device. On the Expo application, shake your physical device to open the "Developer" panel.
Setting up unit and functional tests
To test a React Native application, you can use popular testing tools such as Jest, Enzyme or React Testing Library. These tools let you write unit or functional tests to verify the behavior of your components and functions.
For example, you can write a unit test to check whether a rendered component contains the correct elements:
import React from 'react';
import { render, screen } from '@testing-library/react-native';
import App from './App';
test('Displays the text "Hello world!"', () => {
render();
expect(screen.getByText('Hello world!')).toBeInTheDocument();
});
Publishing and distributing your application
Preparing the production version
Before publishing your React Native application, it's important to make sure it's ready for production. This includes removing any debugging code or sensitive information, as well as checking the application's performance and stability.
It's also a good idea to test your application on different platforms and devices to make sure it works properly in different environments.
APK and IPA file generation
To generate APK and IPA files for your React Native application, you can use the build tools provided by React Native. These tools enable you to generate binary files ready for installation on Android (APK) and iOS (IPA) devices.
To generate an APK file for Android, open your terminal and run the following command:
cd android
./gradlew assembleRelease
The generated APK file will be located in the android/app/build/outputs/apk/release
.
To generate an IPA file for iOS, open your terminal and run the following commands:
cd ios
xcodebuild -workspace MyReactNativeProject.xcworkspace -scheme MyReactNativeProject -archivePath build/MyReactNativeProject.xcarchive archive
xcodebuild -exportArchive -archivePath build/MonProjetReactNative.xcarchive -exportPath build -exportOptionsPlist exportOptions.plist
The generated IPA file will be located in the ios/build
.
Upload to application stores
Once you've generated the APK and IPA files for your React Native app, you can submit them to the respective app stores, Google Play Store and Apple App Storeto make them available for download.
Submitting your app to Google Play Store and Apple App Store involves several steps, including creating a developer account, preparing certain elements such as screenshots and descriptions, and complying with the stores' guidelines and policies.
It's important to review the specific guidelines for each platform and follow the instructions provided by the app stores to successfully submit your app.