Discover the features and potential of Node JS to enhance your web development projects. Learn how to install Node JS and create a server. Discover the advantages of Node JS and the frameworks popular. Harness the power of Node JS for fast, high-performance web applications.
Have you ever wanted to use JavaScript both client-side and server-side? Node JS is the answer. As a JavaScript-based development platform, Node JS enables server-side JavaScript code execution, opening up new possibilities for web developers. In this article, we'll give you a comprehensive introduction to Node JS, exploring its features and potential for enhancing your web development projects.
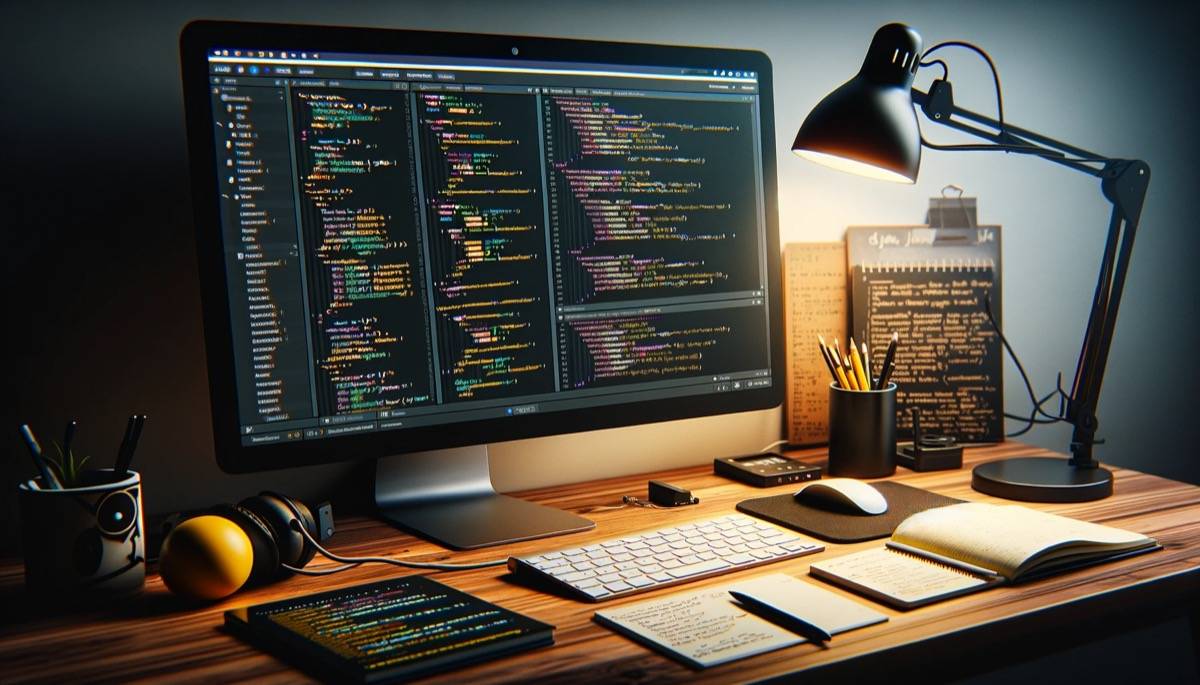
What is Node JS?
A JavaScript runtime environment
Node JS is a JavaScript runtime environment for executing server-side JavaScript code. This means you can use the same programming language on both sides, for both front-end and back-end, facilitating synchronization between the two parts of an application.
Developed by Ryan Dahl
Node JS was developed by Ryan Dahl and its first version was released in 2009. Dahl designed Node JS to meet the need for a fast, high-performance JavaScript execution environment for server-side applications. Since then, Node JS has enjoyed rapid adoption and has become one of the most popular frameworks for web development.
Based on Google's V8 JavaScript engine
Node JS is based on Google’s V8 JavaScript engine, which is also used by the Google Chrome browser. The V8 engine is known for its execution speed and high performance. With this integration, Node JS can benefit from the same high performance, making it an ideal choice for high-performance web applications.
How does Node JS work?
Event model and event loop
Node JS uses an event model and an event loop to manage asynchronous operations. Instead of waiting for each operation to finish before moving on to the next, Node JS uses an event loop to execute multiple operations in parallel. This allows Node JS to be extremely efficient when handling intensive tasks, such as accessing a database or sending HTTP requests.
Non-blocking inputs/outputs
A key feature of Node JS is its support for non-blocking input/output. This means that I/O operations, such as reading or writing files, do not block code execution. With Node JS, you can perform multiple I/O operations at the same time, considerably improving your application's performance.
Using the modules
Node JS supports the use of modules, which are libraries of reusable JavaScript code. Modules allow you to organize your code in a modular way and easily reuse it in different parts of your application. Modules can be downloaded from the Node JS package registry, npm, or you can create your own custom modules.
Advantages of Node JS
High performance
Thanks to its event model and support for non-blocking I/O, Node JS offers high performance. It can efficiently handle multiple simultaneous requests and process intensive tasks without impacting overall application performance. This makes it an excellent choice for high-performance web applications with heavy traffic loads.
Scalability
Node JS is extremely scalable, which means it can easily adapt to the growth of your application. Thanks to its event model and asynchronous nature, Node JS can handle thousands of simultaneous connections with ease. It is also possible to create clusters of nodes (cluster module) to distribute the load and further improve your application's performance.
Ease of development
Node JS uses JavaScript, a popular and widely used programming language, making it very accessible to developers. Many front-end developers are already familiar with JavaScript, which facilitates the transition to Node JS. What's more, Node JS offers numerous libraries and modules that facilitate development, reduce development time and increase developer productivity.
Common uses of Node JS
Real-time web applications
Node JS is particularly well suited to the development of real-time web applications, such as chat applications, real-time dashboards or location tracking systems. Thanks to its event model and asynchronous nature, Node JS can easily handle real-time updates and deliver a fluid, responsive user experience.
Application servers
Node JS is also often used to create application servers. Whether it's for an API, a backend for a mobile application or a web application, Node JS offers an efficient, high-performance solution for managing client requests and supplying the necessary data.
Package building tools
Node JS is often used as a package builder. It is commonly used to manage project dependencies and automate certain tasks, such as merging JavaScript files, compiling CSS files or generating documentation. Using Node JS in this context creates more efficient workflows and saves time for developers.
Install Node JS
Installer download
To install Node JS, you first need to download the installer from the official Node JS website. The installer is available for different operating systems and architectures, so make sure you download the appropriate version for your system.
Installation on different operating systems
Once you've downloaded the installer, you can launch the installation wizard and follow the on-screen instructions to install Node JS on your operating system. Installation is generally straightforward and requires no additional configuration.
Installation check
Once you've installed Node JS, you can check that the installation went smoothly by opening a terminal window and typing the following command:
node -v
This command will display the version of Node JS installed on your system. If you see the version displayed, the installation has been successful.
Creating a server with Node JS
Initiating a project
Before creating a server with Node JS, you must first initialize a project. This is done by creating a folder for your project and executing the following command in a terminal window, inside the project folder:
npm init
This command will guide you through the project initialization process, asking you a few questions about the project name, version, description, and so on. You can also press Enter to accept the default values.
Creating a server file
Once you've initialized the project, you need to create a server file. Create a file with the ".js" extension in your project folder and open it in a text editor. This file will be your server's entry point.
In this file, you need to write JavaScript code to create and configure your server. You can use the built-in "http" module to create a basic HTTP server. Here's an example of code to create a server that listens on port 3000:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Query and response management
Once you've created your server, you can add the logic to manage requests and responses. In the example above, the server's callback function receives two parameters: "req" for the request and "res" for the response.
You can use these parameters to access request information, such as headers or data sent by the client, and to send a response to the client. In the example above, we simply returned the text "Hello, World!" in response to each request.
Module management with Node JS
Using a package manager
Node JS features an integrated package manager called npm (Node Package Manager). npm lets you search for, install and manage JavaScript modules in your project. You can access npm's package registry using the following command in a terminal window, inside your project folder:
npm search module_name
This command will display search results matching the specified module name.
Installing and importing modules
To install a module, you can use the following command in your terminal:
npm install module_name
This command will download and install the specified module in your project's "node_modules" folder.
Once the module is installed, you can import it into your JavaScript code using the "require" function. For example, to import the "express" module, you can use the following line of code:
const express = require('express');
Creating customized modules
As well as using existing modules, you can also create your own custom modules in Node JS. To do this, you need to create a separate JavaScript file containing the code for your module and export it for use in other parts of your application.
Here is an example of a custom module that exports a function to calculate the sum of two numbers:
// myModule.js
function add(a, b) {
return a + b;
}
module.exports = add;
You can then use this custom module in your code by importing it using the "require" function, as follows:
const add = require('./myModule');
const result = add(2, 3);
console.log(result); // Output: 5
Popular frameworks for Node JS
Express.js
Express.js is one of the most popular frameworks for web development with Node JS. It simplifies the process of creating web servers by providing lightweight abstraction for basic functionality such as routing, request and response management, etc. Express.js also boasts a very large developer community and numerous compatible plugins and modules, making it a popular choice for Node JS web projects.
Koa.js
Koa.js is another web framework for Node JS that focuses on flexibility and lightness. Designed by the team behind Express.js, Koa.js offers a more concise syntax and advanced features such as generators and middleware. Koa.js is also highly extensible, meaning you can easily add or remove features as your project requires.
Socket.io
Socket.io is a library that enables real-time communication between server and client. This enables the creation of real-time web applications, such as online chats or real-time dashboards. Socket.io automatically handles low-latency communication and offers a simple API for sending and receiving messages between server and client.
Deploying a Node JS application
Server hosting
To deploy a Node JS application on a server, you must first configure the server to run your application. You can use hosting services such as Amazon Web Services, Microsoft Azure or Google Cloud Platform to deploy your Node JS application. These services offer scalable and flexible hosting solutions for Node JS applications.
You also need to take into account aspects such as dependency management, automatic launch of your application on server startup, error handling and so on. We recommend using tools such as pm2, forever or systemd to manage these aspects when deploying your Node JS application.
Use of cloud services
Another deployment option for Node JS applications is to use cloud services, such as Heroku, AWS Lambda, or Google Cloud Functions. These services offer a ready-to-use infrastructure for running and scaling your Node JS application. You can simply deploy your code on these platforms, and they'll take care of commissioning, scaling, high availability and so on. These services are particularly suitable for lightweight web applications with variable traffic.
Production environment configuration
When deploying a Node JS application in production, it's important to configure your production environment correctly. This includes aspects such as managing environment variables, configuring log files, securing the application and so on.
It is recommended to use environment variables to store sensitive information, such as API keys or database connection information. These environment variables can be retrieved from configuration files or specific deployment tools.
Trends and the future of Node JS
Growing adoption of Node JS
Over the years, Node JS has enjoyed growing adoption among developers and enterprises alike. Its asynchronous nature, high performance and ease of development make it an attractive choice for many projects. Node JS is also used by major companies such as Netflix, LinkedIn and Walmart to power their web applications.
Integration with other technologies
Node JS can be easily integrated with other popular technologies such as React, Angular or Vue.js for the creation of modern web applications. The integration of these technologies enables the creation of responsive and interactive web applications, exploiting the advantages of Node JS on the server side for a better user experience.
Community development
The Node JS community is very active and constantly evolving. New modules, packages and tools are constantly being developed to improve functionality and developer productivity. What's more, the community regularly organizes conferences, hackathons and meetups to share knowledge and experience between community members.
In conclusion, Node JS is a powerful and versatile JavaScript runtime environment for developing fast, high-performance and scalable web applications. Thanks to its event model, non-blocking I/O and wide range of modules and frameworks, Node JS offers significant advantages in terms of performance, scalability and ease of development. Whether for creating real-time web applications, application servers or package building tools, Node JS is an ideal choice for developers wishing to take advantage of server-side JavaScript.