Develop high-performance web applications with React JS. Discover the basics, best practices and installation of React JS. Optimize performance and use components, state and unit tests to create high-performance web applications.
The article "Developing high-performance web applications with React JS" gives you an in-depth look at the use of React JS, a library JavaScriptto create high-performance web applications. With React JS, you can develop user interfaces using isolated, reusable components. This article explores the basic principles of React JS, as well as best practices for optimizing the performance of your web applications. Whether you're an experienced developer or just want to familiarize yourself with this hot technology, this article will provide you with the knowledge you need to create high-performance web applications with React JS.
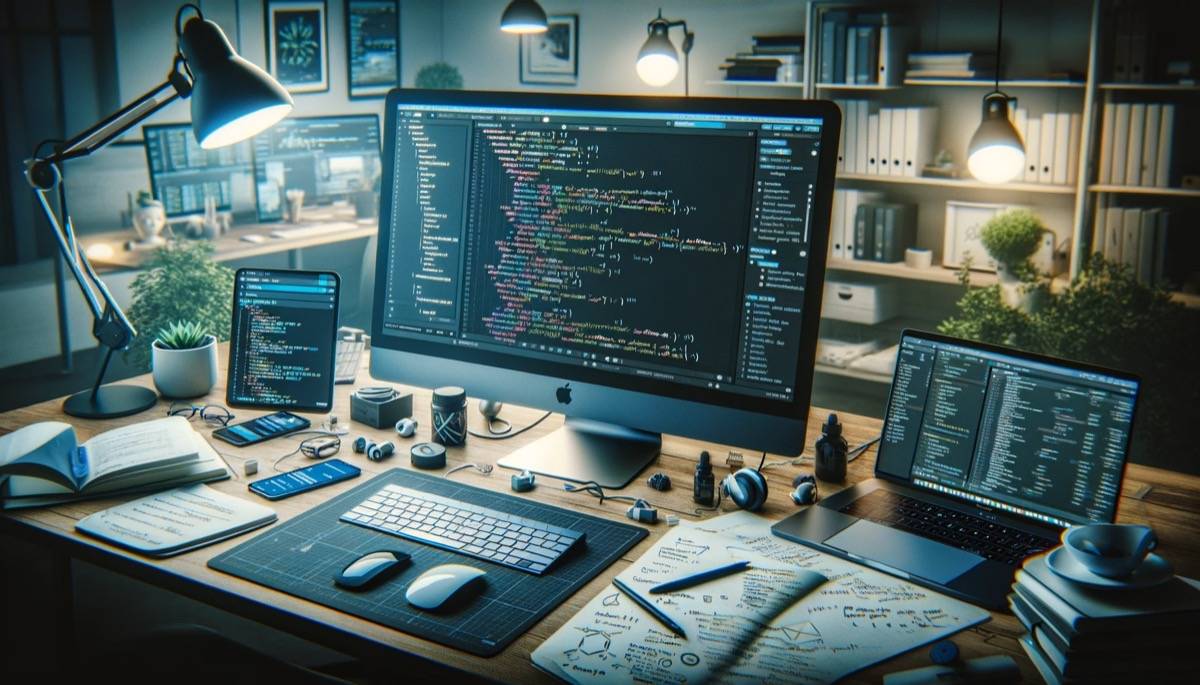
What is React JS
React JS is an open-source JavaScript library used to develop efficient, interactive user interfaces. It was introduced by Facebook in 2013 and is now widely used by developers around the world to create high-performance web applications.
The concept of React JS
The central concept of React JS is the creation of reusable components. A React component is a piece of user interface that can be displayed on the screen and can have its own internal state. These components can be assembled to form complex, interactive user interfaces, greatly facilitating the development of web applications.
Why use React JS
There are many reasons why you should consider using React JS for your web application development. Here are some of the main reasons:
- Optimized performance React JS uses a virtual rendering algorithm that minimizes modifications to the real DOM, making React applications extremely fast and responsive.
- Component reusability Thanks to the modularity of React components, you can easily reuse code and save time when developing new features.
- Easy to test React: React JS makes it easy to create unit tests for your components, so you can improve the quality of your code and detect errors more quickly.
- Rich ecosystem React JS is supported by an active community that offers numerous modules and complementary libraries to extend React's functionality and facilitate development.
Installing React JS
Prerequisites
Before installing React JS, make sure you have the following items:
- Node.js React JS requires Node.js to run its configuration and development files.
- NPM NPM is the Node.js package manager and is used to install and manage React JS dependencies.
Installing React JS
To install React JS, you can use NPM by executing the following command in your terminal:
npm install react
This command will install React JS and all its necessary dependencies. Once the installation is complete, you can start using React JS in your project.
Components in React JS
Component creation
Components are the cornerstone of React JS. They encapsulate the logic and interface of a part of your application and can be reused in several places. Here's how to create a basic component in React JS :
import React from 'react';
class MyComponent extends React.Component {
render() {
return (
My Component
This is an example of a React component.
);
}
}
export default MyComponent;
Using components
Once you've created a component, you can use it in other parts of your application simply by including it in the JSX code. For example, to use the "MyComponent" component created earlier, you could render it like this:
import React from 'react';
import MyComponent from './MyComponent';
class App extends React.Component {
render() {
return (
My Application
);
}
}
export default App;
Communication between components
In a React JS application, components may need to communicate with each other. For this, React JS offers various communication methods, such as passing data via props or using context. These methods enable components to exchange information and update each other according to changes.
State management in React JS
The notion of state in React JS
State is a key concept in React JS. It allows you to store values that can change over time and influence component rendering. In React JS, state is generally managed in class components using the setState()
.
The setState() method
The method setState()
is used to update the state of a component and trigger a new rendering. It takes as parameter an object containing the new state values. Here's an example of how to use the setState()
:
import React from 'react';
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
incrementCount() {
this.setState({
count: this.state.count + 1
});
}
render() {
return (
My Counter: {this.state.count}
);
}
}
export default MyComponent;
Example of report use
In this example, we have a "MyComponent" component that displays a counter and an "Increment" button. When the button is clicked, the incrementCount()
method is called, which updates the counter status using the setState()
. The component automatically updates to reflect the new status value.
Conditional rendering in React JS
Use of conditions
Conditional rendering is an essential feature of React JS, allowing certain elements to be displayed according to a condition. This makes it possible to create dynamic user interfaces that adapt to context. Here's an example of conditional rendering in React JS :
import React from 'react';
class MyComponent extends React.Component {
render() {
const isLoggedIn = this.props.isLoggedIn;
return (
{isLoggedIn ? (
Welcome User
) : (
Welcome Guest
)}
);
}
}
export default MyComponent;
Using ternary operators
Another way to perform conditional rendering in React JS is to use ternary operators. This method is useful when you want to render different elements according to a condition. Here's an example of how to use ternary operators:
import React from 'react';
class MyComponent extends React.Component {
render() {
const isAdmin = this.props.isAdmin;
return (
{isAdmin ? (
Welcome Administrator
) : (
Welcome Standard User
)}
);
}
}
export default MyComponent;
Optimizing performance with React JS
Using the shouldComponentUpdate() method
To optimize the performance of a React JS application, it's important to minimize unnecessary DOM updates. In this context, the shouldComponentUpdate()
can be used to control whether or not a component should be updated. When implemented correctly, this method can help avoid unnecessary rendering and improve your application's performance.
Using the PureComponent method
To facilitate the implementation of performance optimization, React JS offers the component PureComponent
which automatically performs a shallow update check of its props and internal state. This avoids unnecessary rendering when a component's props and state have not changed.
Using React.memo
The function React.memo
is used to store the rendering of a functional component. It is similar to PureComponent
but is used for functional components rather than classes. By wrapping a functional component with React.memo
You can avoid unnecessary rendering by comparing previous and current props.
Routing with React JS
Creating routes with React Router
To manage navigation in a React JS application, you can use the React Router library. It allows you to configure routes for different URLs and render the appropriate components based on the current URL. Here's an example of creating routes with React Router:
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
const Accueil = () =>
Home
;
const Contact = () =>
Contact
;
class App extends React.Component {
render() {
return (
- Home
- Contact
);
}
}
export default App;
Using props for navigation
In React Router, navigation components can use props to pass additional information or perform navigation actions. For example, you can use the history
to redirect to another page. Here's an example of how to use props for navigation:
import React from 'react';
import { withRouter } from 'react-router-dom';
class MyComponent extends React.Component {
handleClick = () => {
this.props.history.push('/next-page');
};
render() {
return (
);
}
}
export default withRouter(MyComponent);
Forms management with React JS
Form field management
Form management in React JS can be handled using state management and events. You can store form field values in the state of a React component and update them using onChange
. Here is an example of how to manage form fields with React JS :
import React from 'react';
class Formulaire extends React.Component {
constructor(props) {
super(props);
this.state = {
name: '',
email: ''
};
}
handleChange = (event) => {
this.setState({
[event.target.name]: event.target.value
});
};
handleSubmit = (event) => {
event.preventDefault();
// Perform actions with form data
};
render() {
return (
);
}
}
export default Form;
Form validation
Form validation is an important step in web application development. For form validation with React JS, you can use validation libraries such as Formik, or simply implement your own validation logic using the setState()
and onChange
.
Unit testing with React JS
Test tools available for React JS
React JS comes with its own testing library called React Testing Library, which provides tools for testing React components. In addition to this, Jest is another commonly used tool for unit testing React JS. These tools enable you to perform unit tests on your React code and ensure that your application works properly.
Creating unit tests for React components
To create unit tests for React components, you can use the tools mentioned above, such as React Testing Library and Jest. You can test component rendering, user interactions, state updates and more. Here's an example of a unit test for a React component:
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import MonComposant from './MonComposant';
test('Counter incrementation test', () => {
const { getByText } = render();
const button = getByText('Increment');
fireEvent.click(button);
expect(getByText('My Counter: 1')).toBeInTheDocument();
});
Good development practices with React JS
Structuring the project
To keep your React JS code clean and easy to manage, it's important to structure your project in an organized way. This can be done by dividing your application into modules and organizing your files according to their functionality. You can also use folders to group together related files such as components, styles and tests.
Using hooks
Hooks are a feature introduced in React JS 16.8 that lets you manage state and other functionality in functional components, without having to convert them to class components. Hooks make it easy to reuse logic between components and simplify code.
Using a CSS framework with React JS
To facilitate the development of user interfaces in React JS, you can use a CSS framework such as Bootstrap or Material-UI. These frameworks provide reusable components and a grid system to help you quickly create an attractive design for your application.